How to Learn React: A Guide for Beginner Web Developers
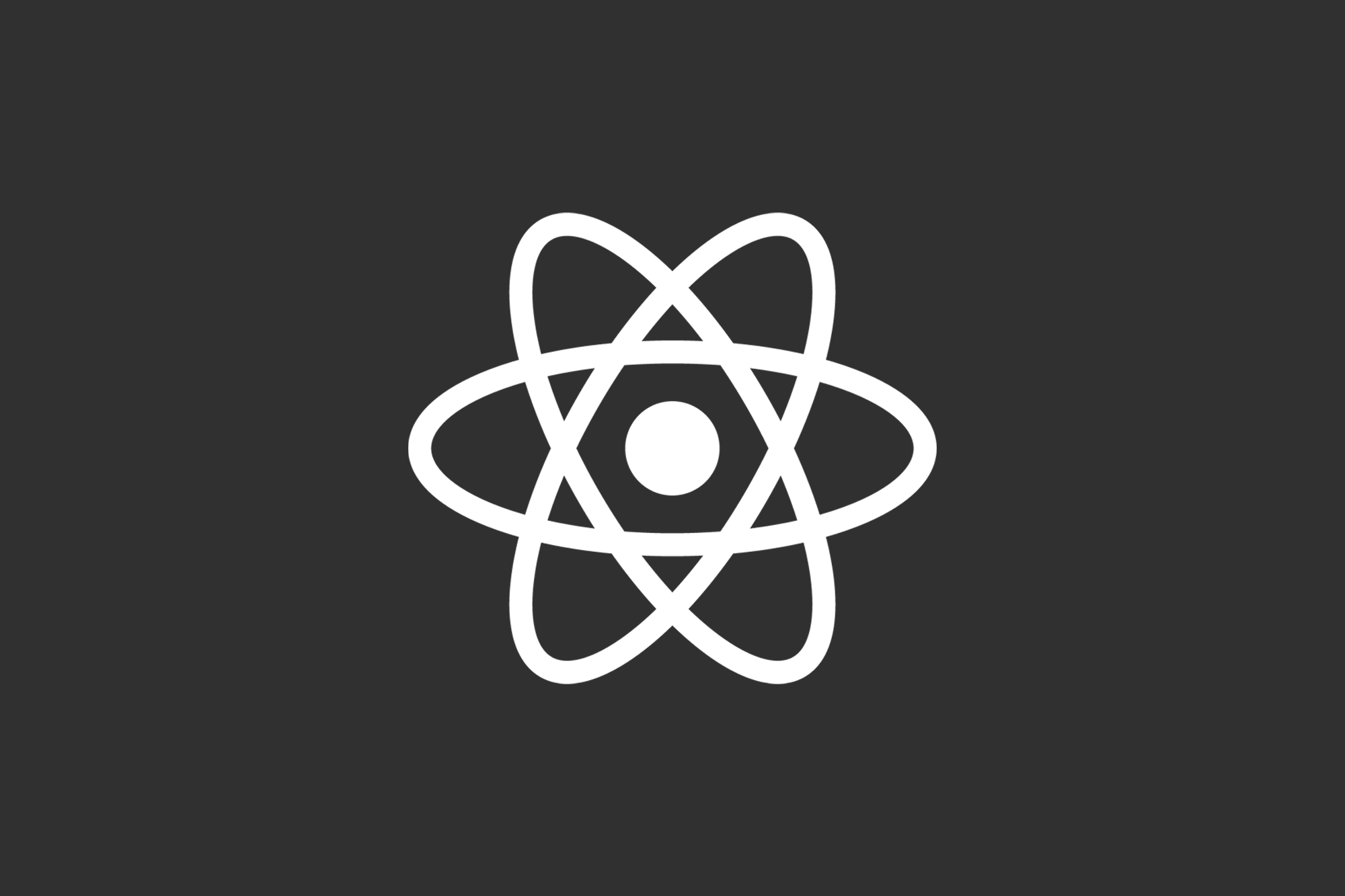
In 2021 Stack Overflow's survey with over 80,000 participating developers, resulted in React pulling ahead of jQuery as the most commonly used web framework. With its declarative, component based API, support for data binding, event and state management - what's not to love? Over nearly a decade React has proven its great benefits. The bi-product of this phenomenon doesn't necessarily benefit new web developers entering the field when considering React's complexity. What the heck is a "component based API", "state management", and "data binding" anyway?
In this post I attempt to break it all down for the beginner web developer to make sense the gobbledygook. The intention of this post is not to provide a deep dive in teaching React, but more about being a guide to help navigate the learning process.
Pointers to Keep in Mind When Learning React
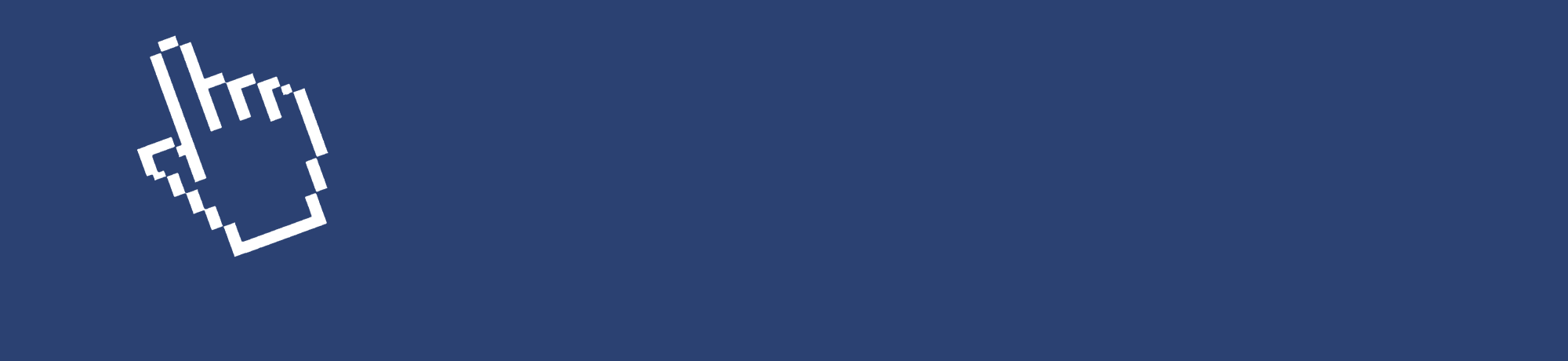
Don't get overwhelmed: once you think you're making sense of React, another term will sideswipe you. My recommendation it to just take it slow, and if you encounter an unrecognized term, pause look it up, and try to make sense of it. Do your best, but if it's still bewildering, move along and save it for another day.
Start simple: React is built to be "unopinionated". It provides a large selection of tools as part of its tool belt and leaves it up to you to pick and choose what to use and how. It provides both simple and advanced concepts to implement. For beginners, I would recommend keeping it simple to start. For example, React Server Components are a new experimental feature being introduced to the library. Before diving into advanced concepts like this, it's important to understand and work with the fundamentals first. You'll need to first understand the difference between "client-side rendering" (or CSR) and "server-side rendering" (SSR). But, don't let all of that scare you... these terms will have their time and place and aren't important when starting off.
Don't combine, mix and match learning: One easy way to burnout before getting started is by piling too much on your plate at once. It's easy to fall into this trap especially when starting a new job that uses a variety of unfamiliar technologies. No job is worth adding unreasonable stress and pressure. Any good job will employ, good, experienced engineers who have a deep understanding of learning curves and the time and patience needed to help grow entry level engineers. It pays off for everyone, by taking it slow. Do not try to learn TypeScript, React and Redux at the same time.
Prerequisites in Learning React
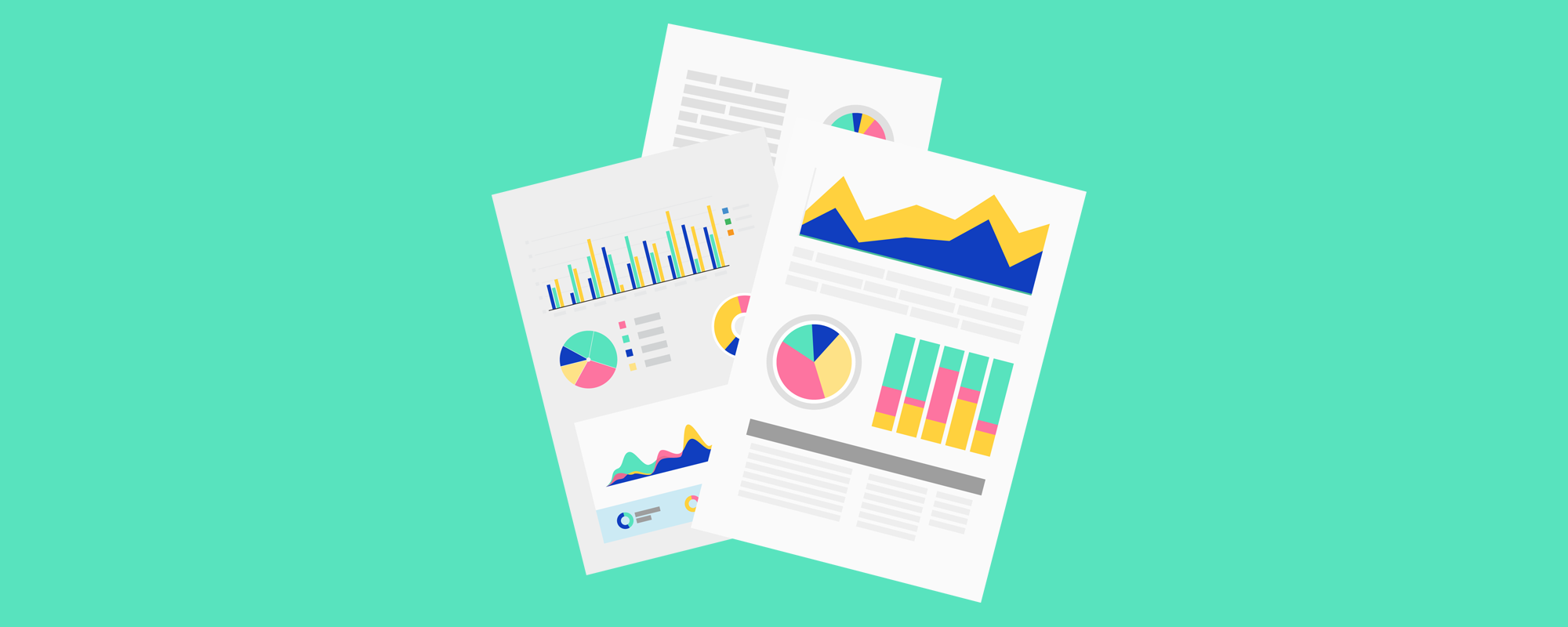
I've worked in the field of web development for nearly two decades and have had the luxury of starting with the fundamentals, not by choice, but simply by nature of the "frontend" web development technology evolution. I was forced to learn certain things before I learned React, because it simply didn't exist. I faced problems in web development first hand that React now solves. I wish new web developers could have this same experience, as its been crucial in my skillset. So, the best recommendation I make to entry level software engineers entering a field or job that uses React is to try to learn the fundamentals of web development first and how React enhances such fundamentals.
Below is the order of operation in learning web development fundamentals that React enhances.
- HTML: React uses "JSX" which is basically an extension of JavaScript that combines HTML-like syntax to support "data-binding". HTML is used in tandem with React. Not only does React "render" components, but also HTML elements. HTML is not especially complicated and it would be wise to establish as much mastery in it as possible... because React, like most component libraries, are outputting a mixture of HTML elements bound by JavaScript functionality.
- CSS: React provides different methods of "styling" and there are variety of tooling around this topic in particular. Before diving into that mess (trust me, it's a messy place historically in React), it would be critical to have a true, deep understanding of how CSS works, in light of all the pre-processors and tooling. Just try writing native CSS and HTML together. Create a barebones
.css
file and add a link to it from your HTML document as an "external stylesheet". Ahhh, the good old days. It's important to learn these basics, so that you know what Webpack and all the other tools typically used with React are doing under the hood. - What is a web page?: There's a lot more than meets the eye here, and before learning React, it's important to just understand the fundamental concepts powering web pages. How is a web page requested and received? How are images, stylesheets, fonts, JavaScript and other files loaded? This will be an on-going learning, but I think it would make sense to take a deeper dive after learning some basic HTML and CSS.
- JavaScript: Well, it's probably a no-brainer JS is on this list. Learning fundamental JavaScript is crucial before learning React, because React is in fact a JavaScript based framework. The key concepts here are around the DOM and how JavaScript can interact with it.
- What is an API (optional): This isn't completely necessary, but you'll find that nearly all React based UIs are communicating in one way or another with an external API... external in a sense that the data is not coming directly from the React UI codebase. The most prominently used and simple API to study is the REST API. GraphQL is another one gaining more traction but much more complicated and not recommended for beginner web developers.
How to Learn React
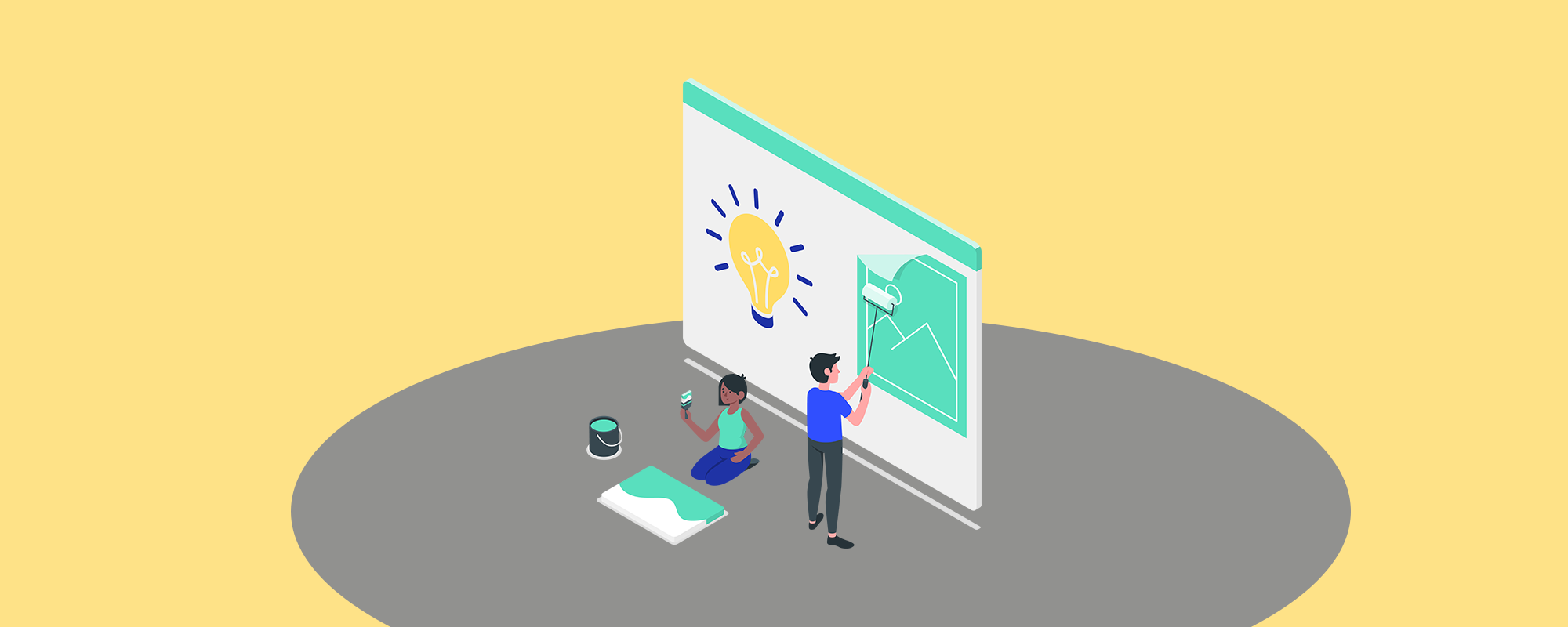
I would strongly recommend learning the above prerequisites before taking a dive into the deep waters of React. Once a establishing at least an amateur level of knowledge in HTML, CSS, and JavaScript, I'd say you're ready for React! Below is how I would recommend learning.
- The Virtual DOM is one of React's unique features. The concept can seem a bit abstract, but if you understand the standard DOM, the Virtual DOM might make more sense. By grasping this concept, one can gain a better understanding of how React code works with the web page.
- What is React and JSX? This would be the natural entry in learning any language or framework, but with React, the answer isn't very straightforward. First, try to wrap your head around the component-driven API. Each component is an encapsulated piece of UI bound by data (
props
and state), represented declaratively. Components are rendered by React into HTML. React has a very complicated reconciliation mechanism, which determines the order in which to render each piece of the tree while accounting for changes in local and global "state" changes... as in changes in data that are bound to the HTML elements... and collections of elements. This component API will make more sense as you learn JSX. - A deeper dive in React: In the old days, components could be written as a JavaScript Class, but nowadays it's more common to use functions. There are tons of features offered by React, but only a handful are used prominently and these are the ones a beginner should focus on:
props
, "hooks" likeuseState
anduseEffect
. - Create an app with "Create React App": It's time to put your learnings to the test! Create React App is great for beginners and in some advanced cases as it simplifies the process of spinning up an app and pre-configures things for most common cases. You can get up and running with one command!
- Learn about build tools like "Webpack": It's important to understand that React isn't running in your browser natively. And there isn't a magical troll converting your code into JavaScript after every change. Nope. The harsh reality is that we have to rely on tools to convert our React code into JavaScript. Webpack is one of the most popular tools for this at the time of this writing.